Video Intelligence (vi)
Video Intelligence component that built into Framework7 allows you to easily add video advertising to your Framework7 app, straight out-of-the-box.
vi's interstitial ads can be placed into your app experience at your discretion, showing full screen video ads. Advertisers pay a premium for these ad slots, and they will be directly connected to your app; you'll be able to monetise straight away.
Getting Started With vi
1. Sign up for Framework7 vi publisher account
First of all as a mobile publisher you need to create vi publisher account. To signup for Framework7 vi account go to vi.framework7.io and fill up the following form:
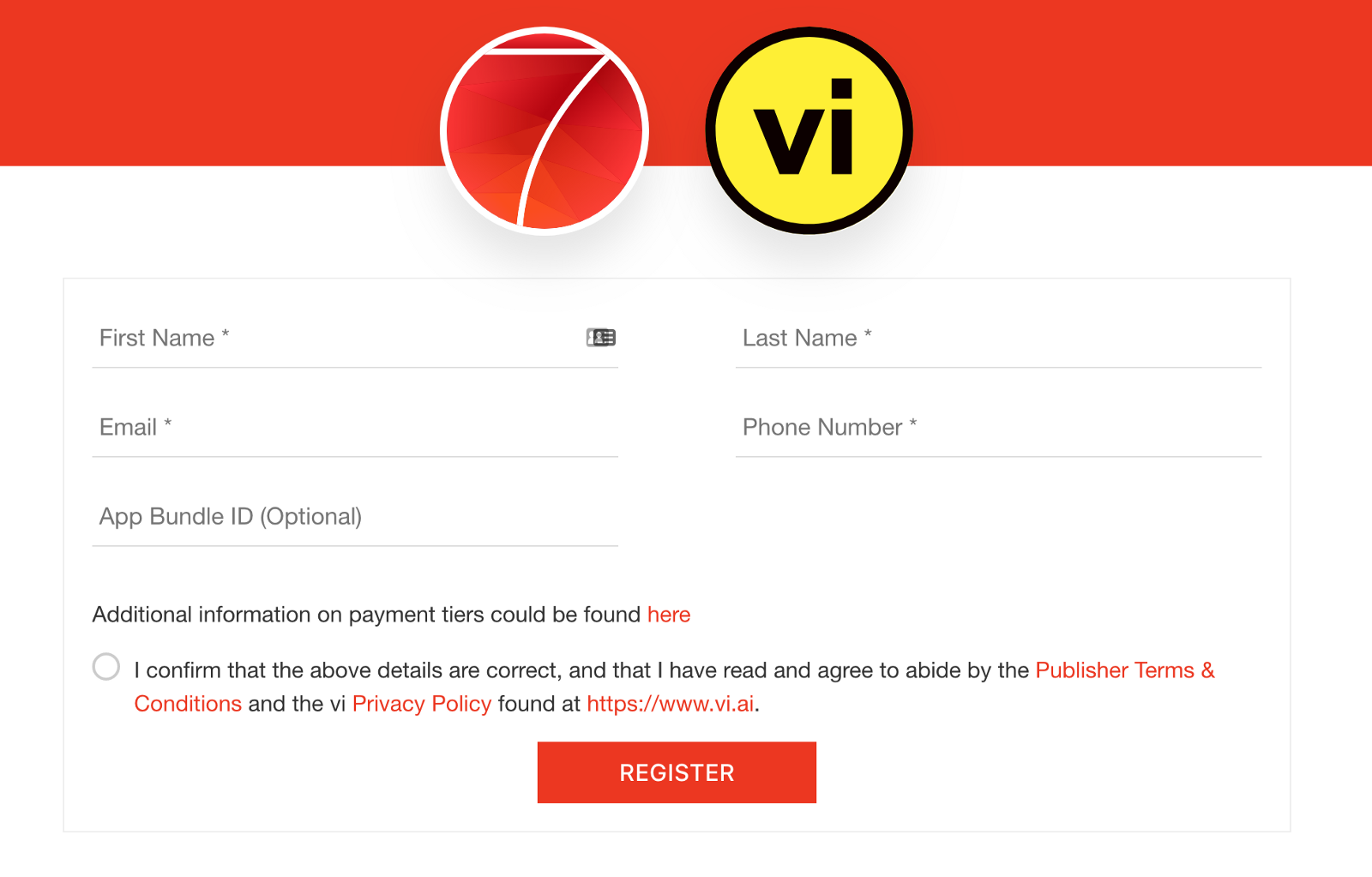
2. Setup your app at vi
After successful registration you will receive instructions with vi dashboard link and temporary password to the specified email.
When you log in to your vi dashboard you will see a list of your apps. If you don’t have apps create new one:

When you already have an app, you need to add placement for this app:

When you create place choose Placement Type: "Video Interstitial"
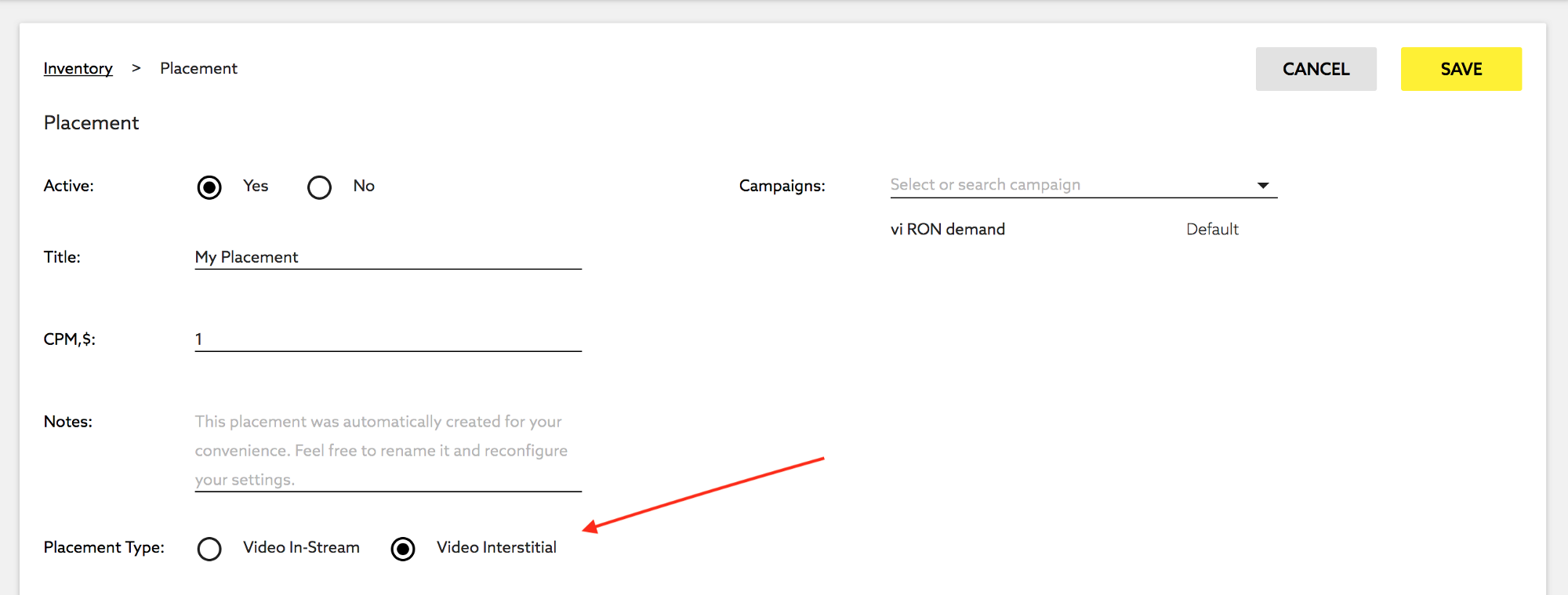
Ok, after we have placement created we need placement ID, choose just created placement:

Click “copy” near the placement id string to copy id to the clipboard
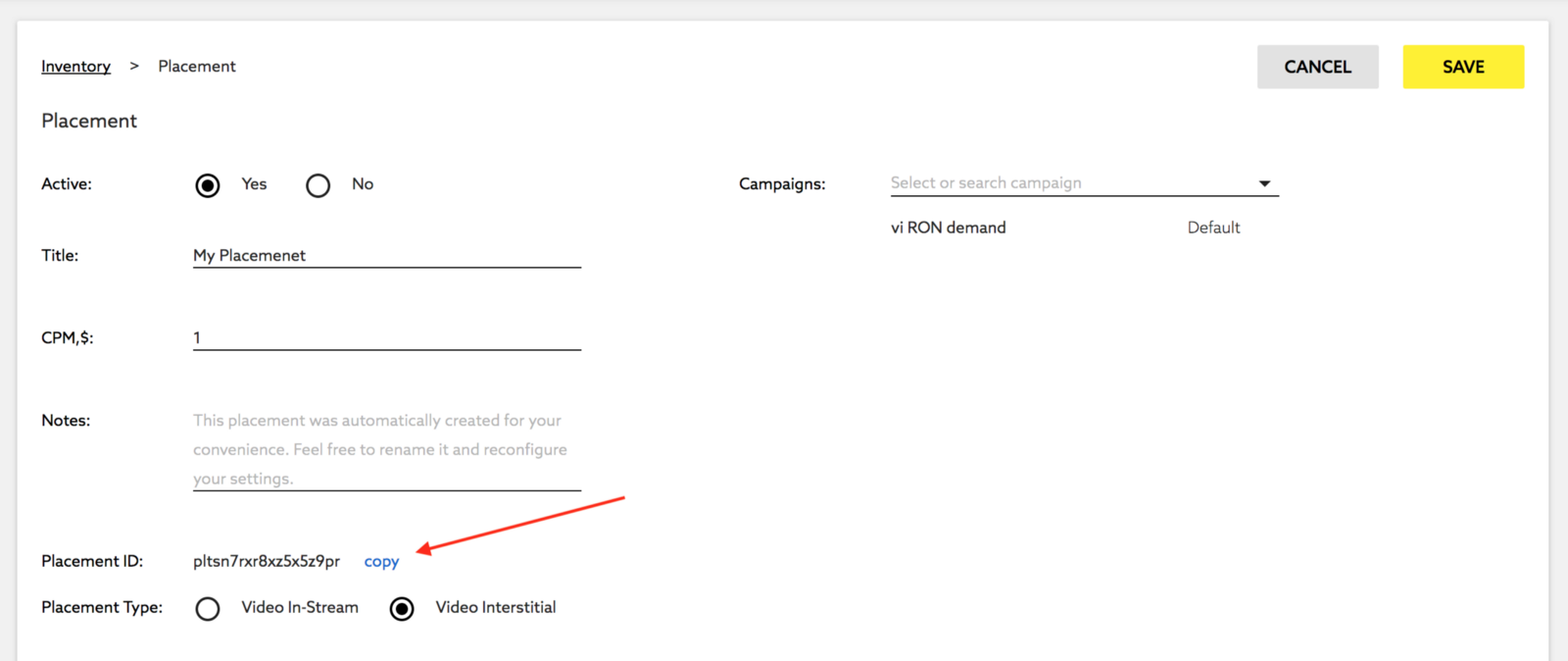
3. Configure vi in Framework7 app
After we set the things up at vi dashboard we will have:
- App bundle id (e.g.
io.framework7.testapp
) - Placement id (e.g.
pltd4o7ibb9rc653x14
)
Now when we have our vi placement id we may set it up in Framework7 on the app initialization:
var app = new Framework7({
/*
common app bundle id must match the one
you have specified when created app in vi dashboard:
*/
id: 'io.framework7.testapp',
/* add vi placement id under the vi module parameters */
vi: {
placementId: 'pltd4o7ibb9rc653x14',
},
});
4. Show Ad
That is all, now we can use Framework7’s vi API to create and show ads. In simplest way just call the following method when you need to show an ad:
app.vi.createAd();
vi App Methods
Let's look at available vi app methods and properties:
app.vi.createAd(parameters) | Create (and show) vi video ad.
|
app.vi.loadSdk() | Loads vi SDK. By default if vi is not disabled in app parameters then it will load it automatically |
app.vi.sdkReady | Boolean property indicating whether the vi SDK is ready or not |
vi Ad Parameters
Let's look at list of all available parameters when we create vi ad:
Parameter | Type | Default | Description |
---|---|---|---|
enabled | boolean | false | Global app parameter which enables vi API and vi SDK to load. If you pass any vi parameters under vi app parameters then it will enable vi api as well |
appId | string | App bundle id, if not specified then equal to id app parameter | |
placementId | string | pltd4o7ibb9rc653x14 | vi placemenet ID, you can use this one for testing, and switch to yours real one for production |
placementType | string | interstitial | Ad type. Can be interstitial (when ad runs as full screen video) or instream (when ad video ) |
autoplay | boolean | true | Enables video ad autoplay |
startMuted | boolean | Defines whether the ad must start muted or not. By default is true when app is running as a web app (not Cordova) under mobile device (iOS or Android). It is required because on mobile device video autoplay usually requires additional user interaction | |
fallbackOverlay | boolean | true | Enables overlay layer that will be visible when ad can not autoplay (in case video autoplay requires user interaction) |
fallbackOverlayText | string | Please watch this ad | Fallback overlay text |
appVer | string | App version, if not specified then equal to version app parameter | |
language | boolean | null | App language, if not specified then equal to language app parameter |
width | number | App width in px. If not specified then equal to app container width | |
height | number | App height in px. If not specified then equal to app container height | |
showProgress | boolean | true | Enable ad interface progress bar |
showBranding | boolean | true | Enable ad interface vi branding logo |
showMute | boolean | true | Enable ad interface mute button |
os | string | Operating system, if not specified then will be equal to app.device.os | |
osVersion | string | Operating system version, if not specified then will be equal to app.device.osVersion | |
orientation | string | Device orientation, if not specified then will be detected based on window.orientation | |
age | number | User age (optional) | |
gender | string | User gender (optional) | |
advertiserId | string | Unique advertiser identifier (optional) | |
latitude | integer | Device location latitude (optional) | |
longitude | integer | Device location longitude (optional) | |
storeId | string | App store id (optional) | |
ip | string | Device IP address (optional) | |
manufacturer | string | Device manufacturer (optional) | |
model | string | Device model | |
connectionType | string | Device connection type (optional) | |
connectionProvider | string | Device connection provider (optional) | |
on | object | Object with events handlers. For example:
|
All parameters can be used in global app parameters under vi
property to set defaults for all vi ads. For example:
var app = new Framework7({
/*
common app bundle id must match the one
you have specified when created app in vi dashboard:
*/
id: 'io.framework7.testapp',
/* global vi parameters */
vi: {
placementId: 'pltd4o7ibb9rc653x14',
}
});
In this case when we create vi ad, we need to pass parameters that we want to override:
var viAd = app.vi.createAd({
// disable autoplay for this ad
autoplay: false,
})
vi Ad Methods & Properties
So to create vi ad we have to call:
var viAd = app.vi.create({ /* parameters */ })
After that we have its initialized instance (like viAd
variable in example above) with useful methods and properties:
Properties | |
---|---|
viAd.app | Link to global app instance |
viAd.ad | Original vi ad instance |
viAd.params | vi ad parameters |
Methods | |
viAd.start() | Start ad video. Useful in case of disabled autoplay |
viAd.pause() | Pause ad video |
viAd.resume() | Resume ad video |
viAd.stop() | Stop ad video, in this case ad will be closed and destroyed. It will be impossible to proceed with this video ad |
viAd.on(event, handler) | Add event handler |
viAd.once(event, handler) | Add event handler that will be removed after it was fired |
viAd.off(event, handler) | Remove event handler |
viAd.off(event) | Remove all handlers for specified event |
viAd.emit(event, ...args) | Fire event on instance |
vi Ad Events
vi ad will fire the following events on vi ad instance:
Event | Target | Arguments | Description |
---|---|---|---|
ready | viAd | Event will be triggered when ad created, requested and ready to be played. In case of disabled autplay, you to listen for this event and call viAd.start() when ad becomes ready | |
started | viAd | Event will be triggered when ad video started to play | |
click | viAd | Event will be triggered after click on ad video | |
click | viAd | url | Event will be triggered after click on ad video. As an argument it received click target URL. Use this url only in case if ad target url can not be opened by your device/app |
impression | viAd | Event will be triggered on ad impression | |
stopped | viAd | reason | Event will be triggered when video ad stopped. As an argument it receives string reason , which can be, for example, userexit (when video was interrupted by user) or complete when ad video completed |
complete | viAd | Event will be triggered when video ad finished | |
userexit | viAd | Event will be triggered when user closed the video ad | |
autoplayFailed | viAd | reason | Event will be triggered in case of enabled autoplay and it failed to start an ad (for example when additional user interraction required) |
error | viAd | error | Event will be triggered on ad error. As an argument it receives error message |
App Events
vi component also fires events on app instance:
Event | Target | Arguments | Description |
---|---|---|---|
viSdkReady | app | Event will be triggered when vi SDK loaded and ready to be used |
Examples
var app = new Framework7({
id: 'io.framework7.testapp',
vi: {
placementId: 'pltd4o7ibb9rc653x14',
}
});
var $$ = Dom7;
// Create prepaire ad
var prepairedAd;
if (!app.vi.sdkReady) {
app.on('viSdkReady', function () {
prepairedAd = app.vi.createAd({
autoplay: false,
});
})
} else {
prepairedAd = app.vi.createAd({
autoplay: false,
});
}
// Show prepaired ad
$$('.show-prepaired').on('click', function () {
prepairedAd.start();
});
// Create and show ad
$$('.create-and-show').on('click', function () {
app.vi.createAd();
});
// With custom autplay fallback
$$('.create-and-show-with-custom-callback').on('click', function () {
app.vi.createAd({
fallbackOverlay: false,
on: {
autoplayFailed: function() {
const ad = this;
app.dialog.alert('Check out this awesome ad', () => {
ad.start();
})
}
}
});
});
// Create and show rewarded ad
$$('.create-and-show-rewarded').on('click', function () {
app.vi.createAd({
autoplay: false,
on: {
ready: function() {
const ad = this;
app.dialog.alert('Check out this awesome ad to get the reward', () => {
ad.start();
})
},
complete: function() {
app.dialog.alert('Congrats! You got the reward!')
},
userexit: function() {
app.dialog.alert('Sorry, no reward for you!')
},
}
});
});